In Java applications (servlets/JSP) users may need to determine absolute or relative paths where files may be uploaded or read from. We show examples for path retrieval and manipulation in Java code.
Physical path (on the server) can be retrieved inside Java/JSP code in many ways. Some of them include:
- fetching path from a System property
- retrieving path using application or context objects
- getting path from a properties file
- hardcode path inside Java/JSP code
- reading path from a paramter in
web.xml
For Java apps the path can be either inside web application root (docRoot
) or somewhere outside of it. Files uploaded to a folder inside web application root (for example to webapps/ROOT/uploads
) will be easily reachable with http://yourdomain.com/uploads/filename. You will need to create an empty subfolder in your project directory before buildng a WAR.
If you choose to save uploaded files outside of Java web application the files will not be reachable directly with an URL. Instead you will need to add some code (usually a servlet) that will fetch the files using Java code and send it to the requesting user.
The 2 cases are shown on the diagram and described below.
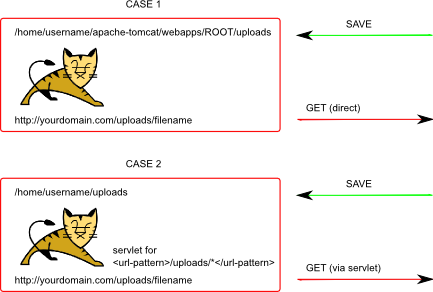
Java JSP servlet get put path
Case 1
We are saving to a subdirectory of Java app web root e.g. /home/username/apache-tomcat/webapps/ROOT/uploads
and fetching directly with http://yourdomain.com/uploads/filename
Case 2
We are saving to a directory above Java app webroot e.g. /home/username/uploads
. We are fetching indirectly with http://yourdomain.com/uploads/filename. Here webapps/ROOT/uploads
does not physically exist. Java code of your app (for example a servlet) is handling /uploads/*
and getting the file from /home/username/uploads/filename
and sending it to the requester.
Here follows a complete JSP code containing a few examples for retrieving absolute path in Java/JSP app and building a new path based upon it.
<%@page import="java.io.*,javax.servlet.*,java.util.Properties" %>
<%
// How to define a path to save data to and/or read data from
// use preexisting system property as base...
String catalinaHome = System.getProperty("catalina.home");
String catalinaBase = System.getProperty("catalina.base");
// ...and build new paths using it
String home = new File(catalinaBase + "/../..").getCanonicalPath();
String subdirOfHome = new File(home + "/audiofiles").getCanonicalPath();
// get current context path
String webRootPath = application.getRealPath("").replace('\\', '/');
// hardcode an absolute or relative path
String hardcodedPath = "/home/username/images";
// specify a path parmeter in web.xml as global context-param
String initPath = getServletContext().getInitParameter("my.context.path");
// define paths in a property file
Properties props = new Properties();
props.load(Thread.currentThread().getContextClassLoader().getResourceAsStream("paths.properties"));
try {
out.println("<b>Examples from JSP</b><br/>");
out.println("Catalina home path: " + catalinaBase + "<br/>");
out.println("Catalina base path: " + catalinaBase + "<br/>");
out.println("Home directory path: " + home + "<br/>");
out.println("Home subdirectory path: " + subdirOfHome + "<br/>");
out.println("Web root path (context): " + webRootPath + "<br/>");
out.println("web.xml param path: " + initPath + "<br/>");
out.println("Hardcoded path: " + hardcodedPath + "<br/>");
out.println("Path read from properties file: "+ props.get("videos") + "<br/>");
out.println("<b>Examples from servlet</b><br/>");
%><jsp:include page="PathServlet" /><%
} catch (Exception e) {
out.println(e.getMessage());
}
%>
PathServlet.java
included in the JSP doGet method follows:
public void doGet(HttpServletRequest request, HttpServletResponse response) throws IOException, ServletException
{
response.setContentType("text/html");
PrintWriter out = response.getWriter();
// specify a path parmeter in web.xml as servlet context-param
String initPath = getServletConfig().getInitParameter("my.servlet.context.path");
String servletRealPath = request.getSession().getServletContext().getRealPath("/");
out.println("web.xml param path (servlet): " + initPath + "<br/>");
out.println("servlet path: " + servletRealPath + "<br/>");
}
Here goes example output of the JSP code displaying differently constructed paths.
For example uploading code see Quick JSP upload tester code using commons-fileupload